n simple words, concurrency is the ability to run several programs or several parts of a program in parallel. Concurrency enable a program to achieve high performance and throughput by utilizing the untapped capabilities of underlying operating system and machine hardware. e.g. modern computers has several CPU’s or several cores within one CPU, program can utilize all cores for some part of processing; thus completing task much before in time in comparison to sequential processing.
The backbone of java concurrency are threads. A thread is a lightweight process which has its own call stack, but can access shared data of other threads in the same process. A Java application runs by default in one process. Within a Java application you can work with many threads to achieve parallel processing or concurrency.
What makes java application concurrent?
The very first class, you will need to make a java class concurrent, is
java.lang.Thread
class. This class is the basis of all concurrency concepts in java. Then you have java.lang.Runnable
interface to abstract the thread behavior out of thread class.
Interview Questions and Answers
tell me name of class in java which is thread-safe?
Stateless Servlet or any stateless class
What is I pass null in synchronized(null) block?
Java synchronization will throw NullPointerException if lock object used in
'synchronized (lock)'
is null
.Object level lock vs Class level lock in Java?
1. Object level lock in Java
Object level lock is mechanism when we want to synchronize a non-static method or non-static code block such that only one thread will be able to execute the code block on given instance of the class. This should always be done to make instance level data thread safe.
2. Class level lock in Java
Class level lock prevents multiple threads to enter in
synchronized
block in any of all available instances of the class on runtime. This means if in runtime there are 100 instances of DemoClass
, then only one thread will be able to execute demoMethod()
in any one of instance at a time, and all other instances will be locked for other threads.
Class level locking should always be done to make static data thread safe. As we know that static keyword associate data of methods to class level, so use locking at static fields or methods to make it on class level.
public class DemoClass { //Method is static public synchronized static void demoMethod(){ } } or public class DemoClass { public void demoMethod() { //Acquire lock on .class reference synchronized (DemoClass. class ) { //other thread safe code } } } or public class DemoClass { private final static Object lock = new Object(); public void demoMethod() { //Lock object is static synchronized (lock) { //other thread safe code } } } |
3. Object level lock vs class level lock – Important notes
- Synchronization in Java guarantees that no two threads can execute a synchronized method, which requires same lock, simultaneously or concurrently.
synchronized
keyword can be used only with methods and code blocks. These methods or blocks can be static or non-static both.- When ever a thread enters into Java
synchronized
method or block it acquires a lock and whenever it leaves synchronized method or block it releases the lock. Lock is released even if thread leaves synchronized method after completion or due to any Error or Exception. - Java
synchronized
keyword is re-entrant in nature it means if a synchronized method calls another synchronized method which requires same lock then current thread which is holding lock can enter into that method without acquiring lock. - Java synchronization will throw
NullPointerException
if object used in synchronized block is null. For example, in above code sample if lock is initialized as null, the “synchronized (lock)
” will throwNullPointerException
. - Synchronized methods in Java put a performance cost on your application. So use synchronization when it is absolutely required. Also, consider using synchronized code blocks for synchronizing only critical section of your code.
- It’s possible that both static synchronized and non static synchronized method can run simultaneously or concurrently because they lock on different object.
- According to the Java language specification you can not use
synchronized
keyword with constructor. It is illegal and result in compilation error. - Do not synchronize on non final field on synchronized block in Java. because reference of non final field may change any time and then different thread might synchronizing on different objects i.e. no synchronization at all.
- Do not use String literals because they might be referenced else where in the application and can cause deadlock. String objects created with
new
keyword can be used safely. But as a best practice, create a new private scopedObject
instance OR lock on the shared variable itself which we want to protect. [Thanks to Anu to point this out in comments.]
Java Inter-thread Communication – PipedReader and PipedWriter?
PipedWriter class is used to write character into stream.
PipedReader class is used to read 1 character from the stream.
now we need to connect them so that 1 thread will write in PipedWriter and
another thread will read from the PipedReader
// Create writer and reader instances
PipedReader pr =
new
PipedReader();
PipedWriter pw =
new
PipedWriter();
// Connect the writer with reader
pw.connect(pr);
Java Deadlock Example and Solution?
1. Deadlock
In Java, a deadlock is a situation where minimum two threads are holding the lock on some different resource, and both are waiting for other’s resource to complete its task. And, none is able to leave the lock on the resource it is holding.
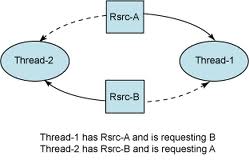
In above case,
Thread-1
has A
but need B
to complete processing and similarly Thread-2
has resource B
but need A
first.package thread; public class ResolveDeadLockTest { public static void main(String[] args) { ResolveDeadLockTest test = new ResolveDeadLockTest(); final A a = test. new A(); final B b = test. new B(); // Thread-1 Runnable block1 = new Runnable() { public void run() { synchronized (a) { try { // Adding delay so that both threads can start trying to // lock resources Thread.sleep( 100 ); } catch (InterruptedException e) { e.printStackTrace(); } // Thread-1 have A but need B also synchronized (b) { System.out.println( "In block 1" ); } } } }; // Thread-2 Runnable block2 = new Runnable() { public void run() { synchronized (b) { // Thread-2 have B but need A also synchronized (a) { System.out.println( "In block 2" ); } } } }; new Thread(block1).start(); new Thread(block2).start(); } // Resource A private class A { private int i = 10 ; public int getI() { return i; } public void setI( int i) { this .i = i; } } // Resource B private class B { private int i = 20 ; public int getI() { return i; } public void setI( int i) { this .i = i; } } } |
Running above code will result in a deadlock for very obvious reasons (explained above). Now we have to solve this issue.
2. How to avoid deadlock
I believe, the solution to any problem lies in identifying the root of the problem. In our case, it is the pattern of accessing the resources
A
and B
, is main issue. So, to solve it, we will simply re-order the statements where the code is accessing shared resources.
i.e both threads should access the common resource in same order.
// Thread-1 Runnable block1 = new Runnable() { public void run() { synchronized (b) { try { // Adding delay so that both threads can start trying to // lock resources Thread.sleep( 100 ); } catch (InterruptedException e) { e.printStackTrace(); } // Thread-1 have B but need A also synchronized (a) { System.out.println( "In block 1" ); } } } }; // Thread-2 Runnable block2 = new Runnable() { public void run() { synchronized (b) { // Thread-2 have B but need A also synchronized (a) { System.out.println( "In block 2" ); } } } }; |
Run again above class, and you will not see any deadlock kind of situation. I hope, it will help you in avoiding deadlocks, and if encountered, in resolving them.
What is AtomicInteger in concurrent package
we will need
AtomicInteger
in two cases:- As an atomic counter which is being used by multiple threads concurrently.
- In compare-and-swap operations to implement non-blocking algorithms.
AtomicInteger atomicInteger =
new
AtomicInteger(
100
);
int
currentValue = atomicInteger.get();
// get the value
atomicInteger.set(
1234
); // set the value
addAndGet()
– Atomically adds the given value to the current value and returns new value after the addition.getAndAdd()
– Atomically adds the given value to the current value and returns old value.incrementAndGet()
– Atomically increments the current value by 1 and returns new value after the increment. It is equivalent to ++i operation.getAndIncrement()
– Atomically increment the current value and returns old value. It is equivalent to i++ operation.decrementAndGet()
– Atomically decrements the current value by 1 and returns new value after the decrement. It is equivalent to i- – operation.getAndDecrement()
– Atomically decrements the current value and returns old value. It is equivalent to – -i operation.
How to Use Locks in Java?
Lock Interface
A
java.util.concurrent.locks.Lock
is a thread synchronization mechanism just like synchronized blocks.
A
Lock
is, however, more flexible and more sophisticated than a synchronized block. Since Lock
is an interface, you need to use one of its implementations to use a Lock in your applications. ReentrantLock
is one such implementation of Lock interface.
Here is the simple use of Lock interface.
Lock lock = new ReentrantLock(); lock.lock(); //critical section lock.unlock(); |
First a Lock is created. Then it’s
lock()
method is called. Now the Lock
instance is locked. Any other thread calling lock()
will be blocked until the thread that locked the lock calls unlock()
. Finally unlock()
is called, and the Lock
is now unlocked so other threads can lock it.Difference between Lock Interface and synchronized keyword
The main differences between a Lock and a synchronized block are:
1) Having a timeout trying to get access to a
synchronized
block is not possible. Using Lock.tryLock(long timeout, TimeUnit timeUnit), it is possible.2) The
synchronized
block must be fully contained within a single method. A Lock can have it’s calls to lock()
and unlock()
in separate methods.
How many ways to create Thread in java?
1. Thread
2. Runnable
3. ThreadFactory
What is ThreadFactory Interface?
1.
ThreadFactory
interface has only one method called newThread()
.
2. It receives a
Runnable
object as a parameter and returns a Thread
object.
3. When you implement a
ThreadFactory
interface, you have to implement that interface and override this method.Java ThreadLocal Variables – When and How to Use?
Difference between Runnable and Callable Interfaces?
ok
0 comments :
Post a Comment