https://www.edureka.co/blog/classloader-in-java/
What is the role for a ClassLoader in Java?
What is JIT in Java? – Understanding Java Fundamentals
use of serialVersionUID in serialization and deserialization in java?
What happens if serialVersionUID is not declared?
If the numbers do not match then, InvalidClassException is thrown. If the loaded class is not having a serialVersionUID declared, then it is automatically generated using the same algorithm as before.
Can 2 classes have same serialVersionUID?
Yes, you can. Serial versions of different classes are independent and do not interfere each other. Eclipse even proposes you to set serialVersionID by default value that is 1L .
Question: Why Constructor in Abstract class?
Answer: Imagine that your abstract class has fields a and b, and that you always want them to be initialized in a certain way, no matter what actual concrete subclass is eventually created.
Question: Can we override static method in Java - Method Hiding
Answer:
1. No, you cannot override static method in Java because method overriding is based upon dynamic binding at runtime and static methods are bonded using static binding at compile time.
2. Though you can create a method with same name and method signature in sub class but that is not overriding. that is actually method hiding in case of static method.
3. Java won't resolve method call at runtime and depending upon type of Object which is used to call static method, corresponding method will be called. It means if you use Parent class's type to call static method, original static will be called from patent class, on ther other hand if you use Child class's type to call static method, method from child class will be called.
Question: What is race condition in Hashmap and how to resolve it.
Difference between hashmap and linkedhashmap?
Internal working of linkedhashmap?
Difference btw fail-fast iterator and fail-safe iterator?
Differnce between Concurrent and Parallel programming?


Answer: Imagine that your abstract class has fields a and b, and that you always want them to be initialized in a certain way, no matter what actual concrete subclass is eventually created.
Question: Can we override static method in Java - Method Hiding
Answer:
1. No, you cannot override static method in Java because method overriding is based upon dynamic binding at runtime and static methods are bonded using static binding at compile time.
2. Though you can create a method with same name and method signature in sub class but that is not overriding. that is actually method hiding in case of static method.
3. Java won't resolve method call at runtime and depending upon type of Object which is used to call static method, corresponding method will be called. It means if you use Parent class's type to call static method, original static will be called from patent class, on ther other hand if you use Child class's type to call static method, method from child class will be called.
public class CanWeOverrideStaticMethod {
public static void main(String args[]) {
Screen scrn = new ColorScreen();
//if we can override static , this should call method from Child class
scrn.show(); //IDE will show warning, static method should be called from classname
}
}
class Screen{
/*
* public static method which can not be overridden in Java
*/
public static void show(){
System.out.printf("Static method from parent class");
}
}
class ColorScreen extends Screen{
/*
* static method of same name and method signature as existed in super
* class, this is not method overriding instead this is called
* method hiding in Java
*/
public static void show(){
System.err.println("Overridden static method in Child Class in Java");
}
}
Output:
Static method from parent class
public static void main(String args[]) {
Screen scrn = new ColorScreen();
//if we can override static , this should call method from Child class
scrn.show(); //IDE will show warning, static method should be called from classname
}
}
class Screen{
/*
* public static method which can not be overridden in Java
*/
public static void show(){
System.out.printf("Static method from parent class");
}
}
class ColorScreen extends Screen{
/*
* static method of same name and method signature as existed in super
* class, this is not method overriding instead this is called
* method hiding in Java
*/
public static void show(){
System.err.println("Overridden static method in Child Class in Java");
}
}
Output:
Static method from parent class
This output confirms that you can not override static method in Java and static method are bonded based upon type information and not based upon Object. had Static mehtod be overridden, method from Child class or ColorScreen would have been called.
HashMap Vs. ConcurrentHashMap Vs. SynchronizedMap – How a HashMap can be Synchronized in Java?
The Map object is an associative containers that store elements, formed by a combination of a uniquely identify
key
and a mapped value
. If you have very highly concurrent application in which you may want to modify or read key value in different threads then it’s ideal to use Concurrent Hashmap. Best example is Producer Consumer which handles concurrent read/write.
So what does the thread-safe Map means? If
multiple threads
access a hash map concurrently, and at least one of the threads modifies the map structurally, it must be synchronized externally
to avoid an inconsistent view of the contents.
How?
There are two ways we could synchronized HashMap
- Java Collections synchronizedMap() method
- Use ConcurrentHashMap
ConcurrentHashMap
- You should use ConcurrentHashMap when you need very high concurrency in your project.
- It is thread safe without synchronizing the
whole map
. - Reads can happen very fast while write is done with a lock.
- There is no locking at the object level.
- The locking is at a much finer granularity at a hashmap bucket level.
- ConcurrentHashMap doesn’t throw a
ConcurrentModificationException
if one thread tries to modify it while another is iterating over it. - ConcurrentHashMap uses multitude of locks.
SynchronizedHashMap
- Synchronization at Object level.
- Every read/write operation needs to acquire lock.
- Locking the entire collection is a performance overhead.
- This essentially gives access to only one thread to the entire map & blocks all the other threads.
- It may cause contention.
- SynchronizedHashMap returns
Iterator
, which fails-fast on concurrent modification.
Difference between hashmap and linkedhashmap?
Internal working of linkedhashmap?
Difference btw fail-fast iterator and fail-safe iterator?
What is a Virtual Function In Java?
The behavior of a virtual function can be overridden with the inheriting class function with the same name. It is basically defined in the base class and overridden in the inherited class.
Virtual function in Java is expected to be defined in the derived class. We can call the virtual function by referring to the object of the derived class using the reference or pointer of the base class.
Every non-static method in Java is by default a virtual method. Java does not have a virtual keyword like C++, but we can define them and use them for concepts like run-time polymorphism.
Virtual Function Example
Let’s take a look at an example to understand how we can use virtual functions in Java.
class Vehicle{ void make(){ System.out.println( "heavy duty" ); } } public class Trucks extends Vehicle{ void make(){ System.out.println( "Transport vehicle for heavy duty" ); } public static void main(String args[]){ Vehicle ob1 = new Trucks(); ob1.make(); } } |
Output: Transport vehicle for heavy duty
Every non-static method in Java is a virtual function except for final and private methods. The methods that cannot be used for the polymorphism is not considered as a virtual function.
A static function is not considered a virtual function because a static method is bound to the class itself. So we cannot call the static method from object name or class for polymorphism. Even when we override the static method it does not resonate with the concept of polymorphism.
Virtual Function With Interfaces
All Java interfaces are virtual, they rely on the implementing classes to provide method implementations. The code for execution is selected at run-time. Here is a simple example for better understanding.
1
2
3
4
5
6
7
8
| interface Car{ void applyBrakes(); } interface Audi implements Car{ void applyBrakes(){ System.out.println( "breaks Applied" ); } } |
Here applyBreaks() is virtual because functions in interfaces are designed to be overridden.
Pure Virtual Function
Pure virtual function is a virtual function for which we don’t have implementations. An abstract method in Java can be considered as a pure virtual function. Let’s take an example to understand this better.
abstract class Dog{ final void bark(){ System.out.println( "woof" ); } abstract void jump(); //this is a pure virtual function } class MyDog extends Dog{ void jump(){ System.out.println( "Jumps in the air" ); } } public class Runner{ public static void main(String args[]){ Dog ob1 = new MyDog(); ob1.jump(); } } |
Output: Jumps in the air
This is how virtual function can be used with abstract class.
Run-Time Polymorphism
Run-time polymorphism is when a call to an overridden method is resolved at run-time instead of compile-time. The overridden method is called through the reference variable of the base class.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
| class Edureka{ public void show(){ System.out.println( "welcome to edureka" ); } } class Course extends Edureka{ public void show(){ System.out.println( "Java Certification Program" ); } public static void main(String args[]){ Edureka ob1 = new Course(); ob1.show(); } } |
Output: Java Certification Course
Points To Remember
- For a virtual function in Java, you do not need an explicit declaration. It is any function that we have in a base class and redefined in the derived class with the same name.
- The base class pointer can be used to refer to the object of the derived class.
- During the execution of the program, the base class pointer is used to call the derived class functions.
This brings us to the end of this article where we have learned about the Virtual Function In Java. I hope you are clear with all that has been shared with you in this tutorial.
What is JIT in Java? – Understanding Java Fundamentals
Every programming language uses a compiler to convert the high-level language code into machine level binary code, because the system understands only the binary code. Based on the type of the programming language, compiler differs. Now talking about the Java Programming language, it uses this amazing compiler called the JIT (Just-in-Time) in Java.
Java JIT Compiler – Overview
The Just-In-Time compiler is one of the integral parts of the Java Runtime Environment. It is mainly responsible for performance optimization of Java-based applications at run time or execution time. In general, the main motto of the compiler is increasing the performance of an application for the end user and the application developer.
Deep Dive into JIT in Java
- Byte code is the chief potential of Java’s WORA (Write once, run anywhere) environment. Speed of the Java application depends on the way the byte code gets converted to the native machine code. The bytecode can either be interpreted or compiled to native code or directly executed on the processor. But, if the bytecode is interpreted, it directly affects the speed of the application.
- In order to speed up the performance, JIT compiler communicates with JVM at the execution time in order to compile byte code sequences into native machine code. Basically, when using JIT Compiler, the native code is easily executed by the hardware when compared to JVM Interpreter. By doing so, there will be a huge gain in execution speed.
- When the JIT Compiler compiles the series of byte code, it also performs certain optimizations such as data analysis, translation from stack operations to register operations, eliminating subexpressions, etc. This makes Java very efficient when it comes to execution and performance.
Now that you know the fundamentals of JIT Compiler, let’s move further and understand its working.
Working of JIT Compiler in Java
The JIT Compiler speeds up the performance of the Java applications at run time. As Java is an object-oriented approach, it comprises of classes and objects. Basically, it constitutes a byte code that is platform independent and executed by the JVM across diversified architectures.
Work Flow:
Below diagram depicts how the actual working of compilation takes place in Java Runtime Environment.
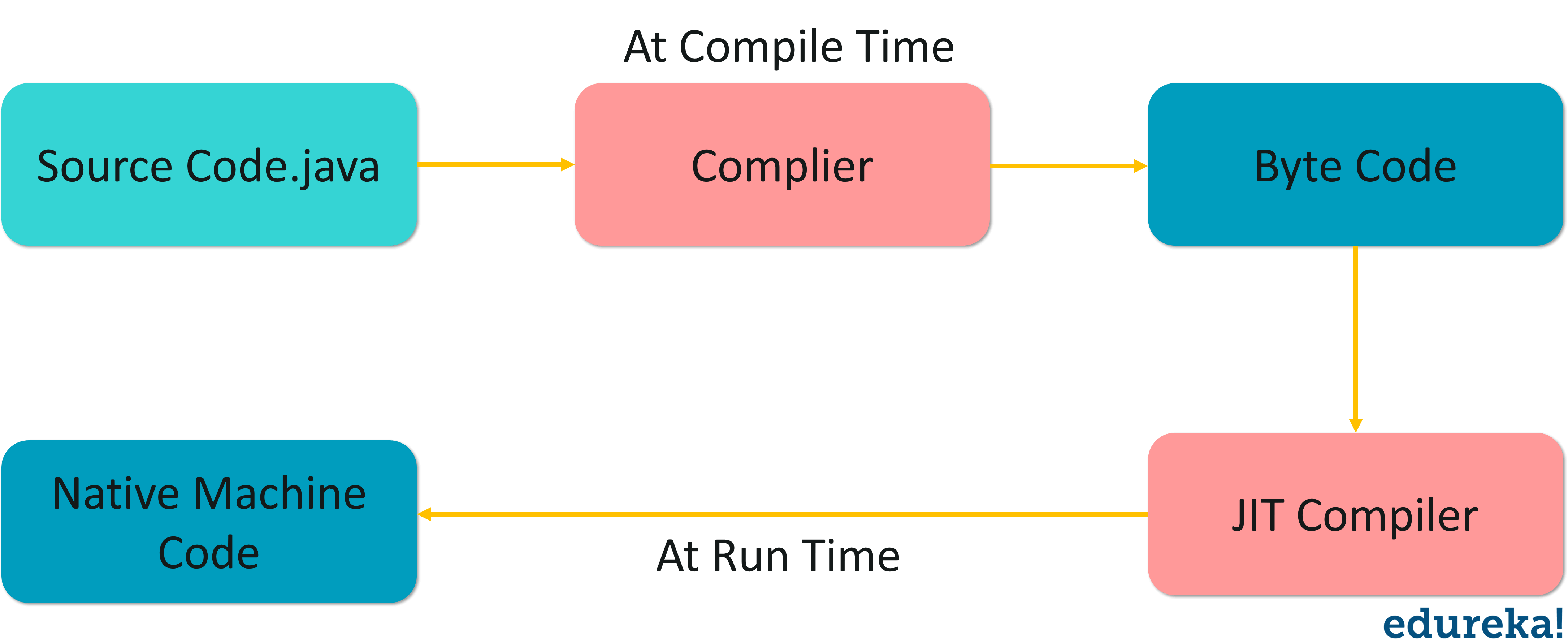
- When you code the Java Program, JRE uses javac compiler to compile the high-level Source code to byte code. After this, JVM loads the byte code at run time and converts into machine level binary code for further execution using Interpreter.
- The JIT Compiler is activated and enabled by default when a method is invoked in Java. When a method is compiled, Java Virtual Machine invokes the compiled code of the method directly without interpreting it. Hence, it does not require much memory usage and processor time. That basically speeds up the performance of the Java Native Application.
So, that’s how it works. Now let’s dive deeper into this article and understand the security aspects of JIT Compiler in Java.
Security Aspects of JIT in Java
The compilation of byte code into machine code by JIT Compiler is done directly in memory. i.e. the compiler feeds the machine code directly into the memory and executes it. In this case, it doesn’t store the machine code into the disk before invoking the class file and executing it. Basically, the memory should be marked as executable. For the sake of security issues, this should be completed after the code is written into the memory. It also should be marked as read-only as executable memory is a security hole. If you wish to know more about this, you can check out this article on JIT Compiler Security aspects.
Now, let’s now move further and know the pros and cons of the Just-In-Time Compiler in Java.
Pros and Cons of JIT in Java
Pros:
- The Java code that you have written years before will run faster even today and that improves the performance of Java Programs.
- Native images also execute faster as they do not possess start-up activities and requires less memory.
Cons:
- Increases the complexity of Java Programs.
- Programs with less code do not benefit with Just-In-Time Compilation.
What is the role for a ClassLoader in Java?
What is a Java Thread Pool and why is it used?
Java EnumSet: How to use EnumSet in Java?
if you want to implement a Set interface with the enumeration type, then you have to use the EnumSet in Java.
EnumSet is an implementation of the Set collections to work with the enum type. EnumSet extends from the AbstractSet and implements the Set interface. The following are a few important points you need to understand about EnumSet in Java:
- Contains only enum values which belong to the same enumeration type
- It is a member of the Java collections framework
- Provides high performance set implementation and is not synchronized
- It does not allow the user to add NULL values and throws a NullPointerException
- Elements are stored in the order of which they are saved
- Uses a fail-safe iteration, which can be used to make sure that ConcurrentModificationException is thrown.
Code Snippet:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
| package edureka; import java.util.ArrayList; import java.util.Collection; import java.util.EnumSet; enum Courses { DevOps, BigData, Python, DataScience, RPA }; public class Example { public static void main(String[] args) { // Create an EnumSet EnumSet<Courses> sample_set; //of method // Add single element sample_set = EnumSet.of(Courses.DevOps); // Display the set System.out.println( "The EnumSet after adding a single element is: " + sample_set); // Add two elements sample_set = EnumSet.of(Courses.DevOps, Courses.BigData); // Display the set System.out.println( "The EnumSet after adding two elements is: " + sample_set); // Add three elements sample_set = EnumSet.of(Courses.DevOps, Courses.BigData, Courses.Python); // Display the set System.out.println( "The EnumSet after adding three elements is: " + sample_set); // Add four elements sample_set = EnumSet.of(Courses.DevOps, Courses.BigData, Courses.Python, Courses.DataScience); // Display the set System.out.println( "The EnumSet after adding four elements is: " + sample_set); // Add five elements sample_set = EnumSet.of(Courses.DevOps, Courses.BigData, Courses.Python, Courses.DataScience,Courses.RPA); // Display the set System.out.println( "The EnumSet after adding five elements is: " + sample_set); //Range method sample_set = EnumSet.range(Courses.BigData, Courses.DataScience); // Display the set System.out.println( "The range of the EnumSet is: " + sample_set); //allOf method sample_set = EnumSet.allOf(Courses. class ); // Display the set System.out.println( "All the elements in the EnumSet are: " + sample_set); //copyOf(Collection) method // Create an empty collection Collection<Courses> samplecollection = new ArrayList<Courses>(); // Add elements to the samplecollection samplecollection.add(Courses.DevOps); samplecollection.add(Courses.BigData); samplecollection.add(Courses.Python); // Display the sample collection set System.out.println( "Elements in the sample collection set are: " + samplecollection); //Create a new EnumSet to store the collection items EnumSet<Courses> final_enumset = EnumSet.copyOf(samplecollection); // Display the EnumSet System.out.println( "Elements in the EnumSet are: " + final_enumset); //copyOf(EnumSet) method // Get all the elements from Courses EnumSet<Courses> example_set = EnumSet.allOf(Courses. class ); // Display the initial EnumSet(sample_set) System.out.println( "The elements in the initial EnumSet are: " + example_set); // Copy the elements from the above set EnumSet<Courses> final_set = EnumSet.copyOf(example_set); // Display the elements in the copied EnumSet System.out.println( "The elements in the copied EnumSet are: " + final_set); //complementOf method //Sample Set sample_set = EnumSet.of(Courses.DevOps, Courses.BigData, Courses.Python); //Create an EnumSet EnumSet<Courses> complement_set; //Complement the above set complement_set = EnumSet.complementOf(sample_set); // Display the elements in the complement EnumSet System.out.println( "The elements in the complement EnumSet are: " + complement_set); //noneOf method // Create empty set EnumSet<Courses> none_example_set = EnumSet.noneOf(Courses. class ); // Display the elements in the set System.out.println( "EnumSet consists of the elements: " + none_example_set); //clone method EnumSet<Courses> final_clone_set = sample_set.clone(); //Display the EnumSet System.out.println( "The clone set consists of the elements:" + final_clone_set); } } |
Concurrency
Concurrency means that an application is making progress on more than one task at the same time (concurrently). Well, if the computer only has one CPU the application may not make progress on more than one task at exactly the same time, but more than one task is being processed at a time inside the application. It does not completely finish one task before it begins the next. Instead, the CPU switches between the different tasks until the tasks are complete.

It is possible to have a concurrent application even though it only has a single thread running inside it. This is one of the goals of our (Nanosai) Thread Ops toolkit, by the way.
Parallelism
Parallelism means that an application splits its tasks up into smaller subtasks which can be processed in parallel, for instance on multiple CPUs at the exact same time.

To achieve true parallelism your application must have more than one thread running, or at least be able to schedule tasks for execution in other threads, processes, CPUs, graphics cards etc.
Concurrency vs. Parallelism In Detail
As you can see, concurrency is related to how an application handles multiple tasks it works on. An application may process one task at at time (sequentially) or work on multiple tasks at the same time (concurrently).
Parallelism on the other hand, is related to how an application handles each individual task. An application may process the task serially from start to end, or split the task up into subtasks which can be completed in parallel.
As you can see, an application can be concurrent, but not parallel. This means that it processes more than one task at the same time, but the thread is only executing on one task at a time. There is no parallel execution of tasks going in parallel threads / CPUs.
An application can also be parallel but not concurrent. This means that the application only works on one task at a time, and this task is broken down into subtasks which can be processed in parallel. However, each task (+ subtask) is completed before the next task is split up and executed in parallel.
Additionally, an application can be neither concurrent nor parallel. This means that it works on only one task at a time, and the task is never broken down into subtasks for parallel execution.
Finally, an application can also be both concurrent and parallel, in that it both works on multiple tasks at the same time, and also breaks each task down into subtasks for parallel execution. However, some of the benefits of concurrency and parallelism may be lost in this scenario, as the CPUs in the computer are already kept reasonably busy with either concurrency or parallelism alone. Combining it may lead to only a small performance gain or even performance loss. Make sure you analyze and measure before you adopt a concurrent parallel model blindly.
What is the purpose of intern() method in the String class?
Answer: It helps in moving the normal string objects to move to the String literal pool
What is the difference between Collections.emptyList() and Collections.EMPTY_LIST?
Answer:
1. Returns the empty list (immutable). This list is serializable.
2. Collections.EMPTY_LIST returns an old-style List
3. Collections.emptyList() uses type-inference and therefore returns List<T>
4. Collections.emptyList() was added in Java 1.5 and it is probably always preferable. This way, you don't need to unnecessarily cast around within your code.
5. In other words, EMPTY_LIST is not type safe:
List list = Collections.EMPTY_LIST;
Set set = Collections.EMPTY_SET;
Map map = Collections.EMPTY_MAP;
As compared to:
List<String> s = Collections.emptyList();
Set<Long> l = Collections.emptySet();
Map<Date, String> d = Collections.emptyMap();
0 comments :
Post a Comment