1. Git is a distributed revision control and source code management software.
2. Git is a free software distributed under the terms of the GNU General Public License version 2.
Version Control System
Version Control System (VCS) is a software that helps software developers to work...
GIT - Tutorial
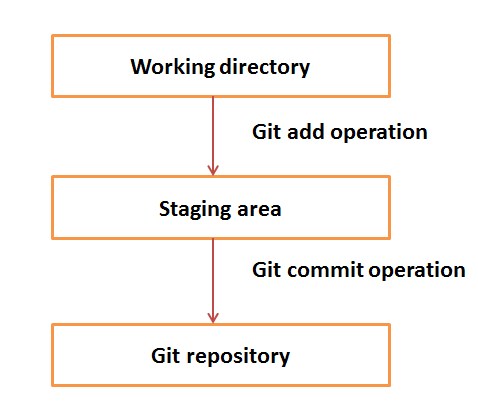